NFKM Functions
This chapter describes the functions and structures that are used in the C NFKM library. This library gives access to Security World key-management functions.
Debugging NFKM functions
Most of the NFKM functions that are described in this chapter can write data to a debug or error log.
However, they do not usually do so except under circumstances outside of those encountered during normal operation (for example, if the module is not properly initialized). You can control the writing of data to a debug or error log with the NFKM_LOG
environment variable.
For more information on the NFKM_LOG
environment variable, see the User Guide.
Use the NFKM_getinfo
call to get the current state before using any other call that relies on the data in the NFKM_SlotInfo
structure being up-to-date.
Functions
Several operations, especially card set creation and loading, require multiple function calls.
In this case there is usually a *_begin
function which must be called first.
There is a *_nextxxx
function that can be called a number of times.
Finally there is a *_done
function.
If, due to user input you decide not to complete the operation there is a *_abort
function which clears up memory.
NFKM_changepp
Change the passphrase on a card.
M_Status NFKM_changepp(
NFast_AppHandle app,
NFastApp_Connection conn,
const NFKM_SlotInfo *slot,
unsigned flags,
const M_Hash *oldpp,
const M_Hash *newpp,
NFKM_ShareFlag remove,
NFKM_ShareFlag set,
struct NFast_Call_Context *cctx
);
-
const NFKM_SlotInfo *slot
is the slot in which the card is loaded -
unsigned flags
is a flags word, the following flag is defined:#define NFKM_changepp_flags_NoPINRecovery 1u
-
const M_Hash *oldpp
is a pointer to the current passphrase hash -
const M_Hash *newpp
is a pointer to the new passphrase hash -
NFKM_ShareFlag remove
is a list of shares whose passphrases you want to remove, regardless of newpp -
NFKM_ShareFlag
set is a list of shares whose passphrases you want to set or change.
The remove and set flags must be disjoint.
A default appropriate to the type of card in the slot is used if both remove and set are zero.
|
NFKM_checkconsistency
This function checks the general consistency of the Security World data:
M_Status NFKM_checkconsistency(
NFast_AppHandle app,
NFKM_DiagnosticContextHandle callctx,
NFKM_diagnostic_callback *informational,
NFKM_diagnostic_callback *warning,
NFKM_diagnostic_callback *fatal,
struct NFast_Call_Context *cctx
);
It returns Status_OK
unless:
-
there was a fatal error, in which case it returns the return value from
fatal()
, which must be nonzero -
any other diagnostic callback returned nonzero, in which case it returns that callback’s return value (because checking was aborted at that point).
NFKM_checkpp
Verifies that a passphrase is correct for a given card. Each share on the card which has a passphrase set is checked.
M_Status NFKM_checkpp(
NFast_AppHandle app,
NFastApp_Connection conn,
const NFKM_SlotInfo *slot,
const M_Hash *pp,
struct NFast_Call_Context *cctx
);
NFKM_cmd_generaterandom
Utility function: calls the nCore GenerateRandom
command.
Requires an app
handle and an existing connection.
M_Status NFKM_cmd_generaterandom(
NFast_AppHandle app,
NFastApp_Connection conn,
M_Word wanted,
unsigned char **block_r,
struct NFast_Call_Context *cctx
);
Sets *block_r
to point to newly allocated memory containing the random data.
NFKM_cmd_destroy
Utility function: calls the nCore Destroy
command to destroy an nCore object.
Requires an app
handle and an existing connection.
M_Status NFKM_cmd_destroy(
NFast_AppHandle app,
NFastApp_Connection conn,
M_ModuleID mn,
M_KeyID idka,
const char *what,
struct NFast_Call_Context *cctx
);
The what
argument should describe what sort of thing you are destroying, for the benefit of people reading log messages created when things go wrong.
NFKM_cmd_loadblob
Utility function: calls the nCore Loadblob
command to load a blob.
Requires an app
handle and an existing connection.
M_Status NFKM_cmd_loadblob(
NFast_AppHandle app,
NFastApp_Connection conn,
M_ModuleID mn,
const M_ByteBlock *blob,
M_KeyID idlt,
M_KeyID *idk_r,
const char *whatfor,
struct NFast_Call_Context *cctx
);
Set idlt
to zero if the blob is module-only.
The whatfor
argument should describe what blob you are loading, for the benefit of people reading log messages created when things go wrong.
NFKM_cmd_getkeyplain
Utility function: calls the nCore Export
command to obtain the plain text of a key object.
Requires an app
handle and an existing connection.
M_Status NFKM_cmd_getkeyplain(
NFast_AppHandle app, NFastApp_Connection
conn,
M_ModuleID mn,
M_KeyID idka,
M_KeyData *keyvalue_r,
const char *what,
struct NFast_Call_Context *cctx
);
The what
argument should describe what sort of key you are querying the plain text of, for the benefit of people reading log messages created when things go wrong.
When you’ve finished with the exported key data, call NFastApp_Free_KeyData
on it.
NFKM_erasecard
This function erases an operator card in the given slot:
M_Status NFKM_erasecard(
NFast_AppHandle app,
NFastApp_Connection conn,
const NFKM_SlotInfo *slot,
NFKM_FIPS140AuthHandle fips140auth,
struct NFast_Call_Context *cctx
);
NFKM_erasemodule
Erases a module. The module must be in (pre-)init mode. All NSO permissions are granted, and the security officer’s key is reset to its default.
M_Status NFKM_erasemodule(
NFast_AppHandle app,
NFastApp_Connection conn,
const NFKM_ModuleInfo *m,
struct NFast_Call_Context *cc
);
-
const NFKM_ModuleInfo *m
is a pointer to the module to be erased.
NFKM_hashpp
This function hashes a passphrase for use as an Operator Card Set passphrase:
M_Status NFKM_hashpp(
NFast_AppHandle app,
NFastApp_Connection conn,
const char *string,
M_Hash *hash_r,
struct NFast_Call_Context *cctx
);
NFKM_initworld_*
NFKM_initworld_abort
Destroys a Security World initialization context.
void NFKM_initworld_abort(
NFKM_InitWorldHandle iwh
);
-
NFKM_InitWorldHandle iwh
is the handle for Security World initialization returned byNFKM_initworld_begin
.
NFKM_initworld_begin
Does the initial part of work for a Security World initialization. The following diagram illustrates the paths through the NFKM_initworld process:
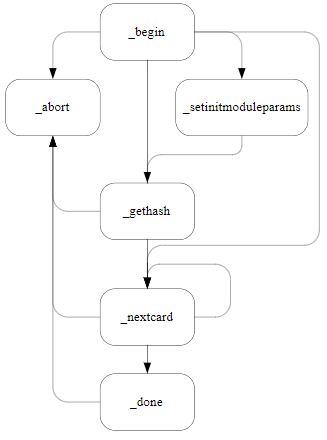
M_Status NFKM_initworld_begin(
NFast_AppHandle app,
NFastApp_Connection conn,
NFKM_InitWorldHandle *iwh,
const NFKM_ModuleInfo *m,
const NFKM_InitWorldParams *iwp,
struct NFast_Call_Context *cc
);
-
NFKM_InitWorldHandle *iwh
is a pointer to the address of handle to set -
const NFKM_ModuleInfo *m
is a pointer to the module to be initialized -
const NFKM_InitWorldParams *iwp
is a pointer to the parameters for new world
If this function fails, nothing will have been allocated and no further action need be taken; if it succeeds, the handle returned must be freed by calling NFKM_initworld_done
or NFKM_initworld_abort
.
It will help if you call NFKM_getinfo
again after this function — otherwise you won’t be able to refer to the module’s slots since it was in PreInitialisation
mode last time you looked.
NFKM_initworld_done
Finishes Security World initialization.
M_Status NFKM_initworld_done(
NFKM_InitWorldHandle iwh
);
-
NFKM_InitWorldHandle iwh
is the handle for Security World initialization returned byNFKM_initworld_begin
.
If this function succeeds, the handle will have been freed; if it fails, you must still call NFKM_initworld_abort
.
NFKM_initworld_gethash
Fetches the identifying hash for new administrator cards created by this job.
void NFKM_initworld_gethash(
NFKM_InitWorldHandle iwh,
M_Hash *hh
);
-
NFKM_InitWorldHandle iwh
is the handle for Security World initialization returned byNFKM_initworld_begin
. -
M_Hash *hh
is a pointer to a memory location to which you want the function to write the hash
NFKM_initworld_nextcard
Writes an administrator card.
M_Status NFKM_initworld_nextcard(
NFKM_InitWorldHandle iwh,
NFKM_SlotInfo *s,
const M_Hash *pp,
int *left
);
-
NFKM_InitWorldHandle iwh
is the handle for Security World initialization returned byNFKM_initworld_begin
. -
NFKM_SlotInfo *s
is a pointer to the slot containing the admin card -
const M_Hash *pp
is a pointer to the passphrase for the card -
int *left
is the address to store number of cards remaining.
NFKM_initworld_setinitmoduleparams
Configures the parameters for module initialization at the end of the world initialization.
M_Status NFKM_initworld_setinitmoduleparams(
NFKM_InitWorldHandle iwh,
const NFKM_InitModuleParams *imp
);
-
NFKM_InitWorldHandle iwh
is the handle for Security World initialization returned byNFKM_initworld_begin
. -
const NFKM_InitModuleParams *imp
is a pointer to the module initialization params.
NFKM_loadadminkeys_*
NFKM_loadadminkeys_begin
Initializes an operation to load administrator keys.
Initially, no tokens are selected for loading.
The following diagram illustrates the paths through the NFKM_loadadminkeys
process:
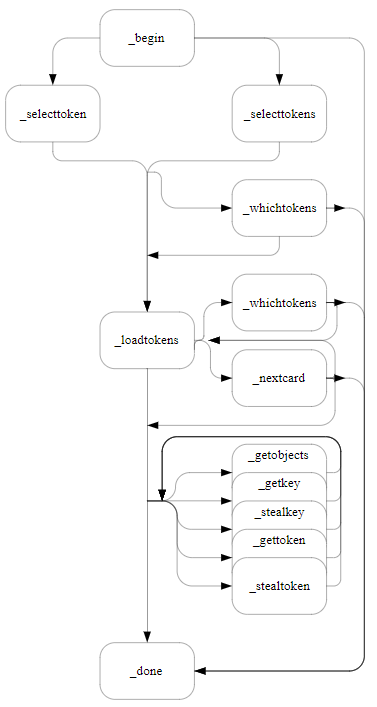
M_Status NFKM_loadadminkeys_begin(
NFast_AppHandle app,
NFastApp_Connection conn,
NFKM_LoadAdminKeysHandle *lakh,
const NFKM_ModuleInfo *m,
struct NFast_Call_Context *cc
);
-
NFKM_LoadAdminKeysHandle *lakh
is a pointer to the address to which the function writes a handle for this operation. -
const NFKM_ModuleInfo *m
is a pointer to the module on which you wish to load the keys.
NFKM_loadadminkeys_done
Frees a key loading context. Any keys and tokens remaining owned by the context are destroyed.
void NFKM_loadadminkeys_done( NFKM_LoadAdminKeysHandle lakh );
-
NFKM_LoadAdminKeysHandle lakh
is the handle returned byNFKM_loadadminkeys_begin
NFKM_loadadminkeys_{get,steal}{key,token}
These are convenience functions which offer slightly simpler interfaces than NFKM_loadadminkeys_getobjects
.
The steal
functions set the NFKM_LAKF_STEAL
flag, which the get
functions do not; the key
functions load keys whereas the token
functions fetch logical tokens.
See NFKM_loadadminkeys_getobjects
for full details about the behavior of these functions.
M_Status NFKM_loadadminkeys_getkey(
NFKM_LoadAdminKeysHandle lakh,
int i,
M_KeyID *k
);
M_Status NFKM_loadadminkeys_stealkey(
NFKM_LoadAdminKeysHandle lakh,
int i,
M_KeyID *k
);
M_Status NFKM_loadadminkeys_gettoken(
NFKM_LoadAdminKeysHandle lakh,
int i,
M_KeyID *k
);
M_Status NFKM_loadadminkeys_stealtoken(
NFKM_LoadAdminKeysHandle lakh,
int i,
M_KeyID *k
);
-
NFKM_LoadAdminKeysHandle lakh
is the handle returned byNFKM_loadadminkeys_begin
-
int i
is the label for the key or token -
M_KeyID *k
is a pointer to the address to store the keyid
A key cannot be loaded once its logical token has been stolen. Therefore, if you want to steal a key and its token, you must steal the key first. |
NFKM_loadadminkeys_getobjects
Extracts objects from the admin keys
context.
M_Status NFKM_loadadminkeys_getobjects(
NFKM_LoadAdminKeysHandle lakh,
M_KeyID *v,
const int *v_k,
const int *v_lt,
unsigned f
);
-
NFKM_LoadAdminKeysHandle lakh
is the handle returned byNFKM_loadadminkeys_begin
-
M_KeyID *v
is a pointer to the output vector of keyids -
const int *v_k
is a vector of key labels -
const int *v_lt
is a vector of token labels -
unsigned f
is a bitmap of flags
Extracts objects from the admin keys context.
Logical tokens must have been loaded using the selecttokens
, loadtokens
and nextcard
interface; keys must have their protecting logical token loaded already.
The KeyIDs
for the objects are stored in the array v
in the order of their labels in the v_k
and v_lt
vectors, keys first.
The label vectors are terminated by an entry with the value -1. Either v_k
or v_lt
(or both) may be null to indicate that no objects of that type should be loaded.
Usually, the context retains ownership of the objects extracted: the objects will remain available to other callers, and will be Destroyed when the context is freed.
If the flag NFKM_LAKF_STEAL
is set in f
, the context will forget about the object; it will not be available to subsequent callers, nor be Destroyed automatically.
Stealing a logical token will prevent keys from being loaded from blobs until that token is reloaded. However, note that keys which have already been loaded but not stolen will remain available. |
As an example, consider the case where LT
R has been loaded.
Two calls are made to getobjects: one which fetches K
RE, and a second which steals the token LT
R. It is no longer possible to get K
RA (because LT
R is now unavailable), but further requests to get K
RE will be honoured.
If an error occurs, the contents of the vector v
are unspecified, and no objects will have been stolen.
However, some of the requested keys may have been loaded.
NFKM_loadadminkeys_loadtokens
Starts loading the necessary tokens.
It might be possible that they’re all loaded already, in which case *left
is reset to zero on exit.
M_Status NFKM_loadadminkeys_loadtokens(
NFKM_LoadAdminKeysHandle lakh,
int *left
);
-
NFKM_LoadAdminKeysHandle lakh
is the handle returned byNFKM_loadadminkeys_begin
-
int *left
is the address at which to store the number of cards remaining.
NFKM_loadadminkeys_nextcard
Reads an admin card.
M_Status NFKM_loadadminkeys_nextcard(
NFKM_LoadAdminKeysHandle lakh,
const NFKM_SlotInfo *s,
const M_Hash *pp, int *left
);
-
NFKM_LoadAdminKeysHandle lakh
is the handle returned byNFKM_loadadminkeys_begin
-
const NFKM_SlotInfo *s
is a pointer to slot to read -
const M_Hash *pp
is a pointer to passphrase hash, orNULL
if the card has no passphrase -
int *left
is the address at which to store the number of cards remaining.
NFKM_loadadminkeys_selecttoken
Selects a single token to be loaded.
M_Status NFKM_loadadminkeys_selecttokens(
NFKM_LoadAdminKeysHandle lakh,
int k
);
-
NFKM_LoadAdminKeysHandle lakh
is the handle returned byNFKM_loadadminkeys_begin
-
int *k
is a key or token label. A key label requests that the token protecting that key be loaded.
NFKM_loadadminkeys_selecttokens
Selects a collection of tokens to be loaded.
M_Status NFKM_loadadminkeys_selecttokens(
NFKM_LoadAdminKeysHandle lakh,
const int *k
);
-
NFKM_LoadAdminKeysHandle lakh
is the handle returned byNFKM_loadadminkeys_begin
-
const int *k
is an array of key or token labels
The array is terminated by an entry containing the value -1. Each entry may be either a key or token label. A key label requests that the token protecting that key be loaded.
NFKM_loadadminkeys_whichtokens
Discovers which logical tokens will be read in the next or current loadtokens
operation.
NFKM_ShareFlag NFKM_loadadminkeys_whichtokens(
NFKM_LoadAdminKeysHandle lakh
);
-
NFKM_LoadAdminKeysHandle lakh
is the handle returned byNFKM_loadadminkeys_begin
Returns a bitmap of logical tokens to be loaded.
NFKM_loadcardset_*
NFKM_loadcardset_abort
This function aborts the loading of a card set:
void NFKM_loadcardset_abort(
NFKM_LoadCSHandle state
);
NFKM_loadcardset_begin
Use the NFKM_getinfo call to get the current state before using any other call that relies on the data in the NFKM_SlotInfo structure being up to date.
|
This function prepares to load a card set.
The following diagram illustrates the paths through the NFKM_loadcardset process
:
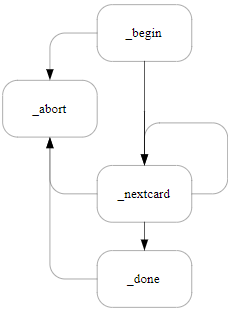
M_Status NFKM_loadcardset_begin(
NFast_AppHandle app,
NFastApp_Connection conn,
const NFKM_ModuleInfo *module,
const NFKM_CardSet *cardset,
NFKM_LoadCSHandle *state_r,
struct NFast_Call_Context *cctx
);
NFKM_loadcardset_done
This function completes the loading of a card set:
M_Status NFKM_loadcardset_done(
NFKM_LoadCSHandle state,
M_KeyID *logtokid_r
);
NFKM_loadcardset_nextcard
Use the NFKM_getinfo call to get the current state before using any other call that relies on the data in the NFKM_SlotInfo structure being up to date.
|
This function attempts to load the next card in a card set:
M_Status NFKM_loadcardset_nextcard(
NFKM_LoadCSHandle state,
const NFKM_SlotInfo *slot,
const M_Hash *pp,
int *sharesleft_r,
struct NFast_Call_Context *cctx
);
It returns Status_OK
if the card was loaded successfully.
Otherwise, in the event of an error, the return value will be TokenIOError
, PhysTokenNotPresent
, DecryptFailed
, or potentially something else in the event of an unrecoverable error.
After any error, even a recoverable one, *sharesleft_r
is not changed.
NFKM_loadworld_*
NFKM_loadworld_abort
Destroys a Security World loading context.
void NFKM_loadworld_abort(
NFKM_LoadWorldHandle lwh
);
-
NFKM_LoadWorldHandle lwh
is the handle for the Security World to be loaded returned byNFKM_loadworld_begin
.
NFKM_loadworld_begin
Initializes an operation to program a module with an existing Security World.
The following diagram illustrates the paths through the NFKM_loadworld
process:
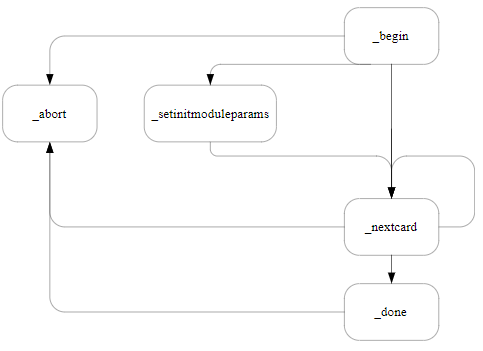
M_Status NFKM_loadworld_begin(
NFast_AppHandle app,
NFastApp_Connection conn,
NFKM_LoadWorldHandle *lwh,
const NFKM_ModuleInfo *m,
struct NFast_Call_Context *cc
);
-
NFKM_LoadWorldHandle *lwh
is a pointer to the address of handle to fill in -
const NFKM_ModuleInfo *m
is a pointer to the module to be initialized
If this function fails, nothing will have been allocated and no further action need be taken; if it succeeds, the handle returned must be freed by calling NFKM_loadworld_done
or NFKM_loadworld_abort
.
As with initializing new Security Worlds, it will help if you call NFKM_getinfo
again after this function.
NFKM_loadworld_done
Finishes Security World loading.
M_Status NFKM_loadworld_done(
NFKM_LoadWorldHandle lwh
);
-
NFKM_LoadWorldHandle lwh
is the handle for the Security World to be loaded returned byNFKM_loadworld_begin
.
If this function succeeds, the handle will have been freed; if it fails, you must still call NFKM_loadworld_abort
.
NFKM_loadworld_nextcard
Reads an administrator card.
M_Status NFKM_loadworld_nextcard(
NFKM_LoadWorldHandle lwh,
const NFKM_SlotInfo *s,
const M_Hash *pp, int *left
);
-
NFKM_LoadWorldHandle lwh
is the handle for the Security World to be loaded returned byNFKM_loadworld_begin
. -
const NFKM_SlotInfo *s
is a pointer to the slot containing the admin card -
const M_Hash *pp
is a pointer to the passphrase for the card -
int *left
is a pointer to the address to store number of cards remaining
NFKM_loadworld_setinitmoduleparams
Configures the parameters for module initialization at the end of the world initialization.
M_Status NFKM_loadworld_setinitmoduleparams(
NFKM_LoadWorldHandle lwh,
const NFKM_InitModuleParams *imp
);
-
NFKM_LoadWorldHandle lwh
is the handle for the Security World to be loaded returned byNFKM_loadworld_begin
-
const NFKM_InitModuleParams *imp
is a pointer to the module initialization parameters.
NFKM_makecardset_*
NFKM_makecardset_abort
This function aborts the creation of a card set:
void NFKM_makecardset_abort(
NFKM_MakeCSHandle state
);
NFKM_makecardset_begin
This function prepares to make a new card set.
The following diagram illustrates the paths through the NFKM_makecardset
:
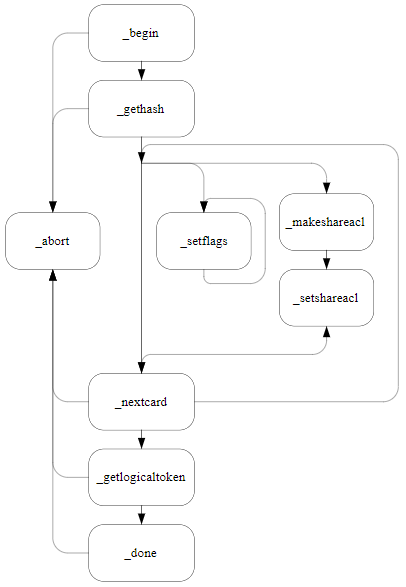
NFKM_makecardset_setflags , NFKM_makecardset_makeshareacl or NFKM_makecardset_setshareacl are not recommended for normal use
|
Use the NFKM_getinfo call to get the current state before using any other call that relies on the data in the NFKM_SlotInfo structure being up to date.
|
M_Status NFKM_makecardset_begin(
NFast_AppHandle app,
NFastApp_Connection conn,
const NFKM_ModuleInfo *module,
NFKM_MakeCSHandle *state_r,
const char *name,
int n,
int k,
M_Word flags,
int timeout,
NFKM_FIPS140AuthHandle fips140auth,
struct NFast_Call_Context *cctx
);
-
const NFKM_ModuleInfo *module
is a pointer to the module to use to make the card set -
NFKM_MakeCSHandle *state_r
is a pointer to the card set state.
typedef struct NFKM_MakeCSState
*NFKM_MakeCSHandle;
-
const char *name
is the name to use for this card set. -
int n
is the total number of cards in the set -
int k
is the the quorum, the number of cards that must be read to recreate the logical token. -
M_Word
flags a flags word, the following flag is defined:
NFKM_SAF_REMOTE 1u /*Allow remote reading of shares */
-
int timeout
is the time out for the card set or 0 for no time out. This is the time in seconds from the loading of the card set after which the module will destroy the logical tokens protected by the card set.
NFKM_FIPS140AuthHandle fips140auth
is only required in FIPS 140 Level 3 Security Worlds.
NFKM_makecardset_done
This function completes the creation of a card set:
M_Status NFKM_makecardset_done(
NFKM_MakeCSHandle state,
NFKM_CardSetIdent *ident_r,
NFKM_FIPS140AuthHandle fips140auth
);
NFKM_makecardset_gethash
The functions fetches the identifying hash for cards created by this makecardset
job.
void NFKM_makecardset_gethash(
NFKM_MakeCSHandle mch,
M_Hash *hh
);
NFKM_makecardset_getlogicaltoken
Fetches the logical token id for a card set which has been written.
M_Status NFKM_makecardset_getlogicaltoken(
NFKM_MakeCSHandle mch,
M_KeyID *ltid,
unsigned f
);
#define NFKM_MCF_STEAL 1u
Only call this function after NFKM_makecardset_nextcard
says there are no shares left.
If you set NFKM_MCF_STEAL
in f
then you get to keep the logical token id and NFKM_makecardset_done
won’t destroy it.
NFKM_makecardset_makeshareacl
Constructs a share ACL.
M_Status NFKM_makecardset_makeshareacl(
NFKM_MakeCSHandle mch,
M_Word f,
M_ACL *acl
);
Dispose of the ACL using NFastApp_FreeACL
when you’ve finished.
NFKM_makecardset_nextcard
Use the NFKM_getinfo call to get the current state before using any other call that relies on the data in the NFKM_SlotInfo structure being up to date.
|
This function writes the next card in a new card set:
M_Status NFKM_makecardset_nextcard(
NFKM_MakeCSHandle state,
const char *name,
NFKM_SlotInfo *slot,
const M_Hash *pp,
int *sharesleft_r,
NFKM_FIPS140AuthHandle fips140auth
);
It returns values and semantics as for NFKM_loadcardset_nextcard
.
The per-card name must be NULL
for n=1
card sets, and non-NULL
for all other card sets.
NFKM_makecardset_setflags
M_Word NFKM_makecardset_setflags(
NFKM_MakeCSHandle mch,
M_Word bic,
M_Word xor
);
Returns the current flags; then clears the bits in bic
and toggles the bits in xor
.
The flags wanted are the Card_flags_*
ones.
It is best to avoid using this function; instead, pass appropriate CardSet_flags_ to NFKM_makecardset_begin and it will automatically set appropriate share flags.
|
NFKM_makecardset_setshareacl
Sets the ACL to be set on subsequent shares of this card set.
void NFKM_makecardset_setshareacl(
NFKM_MakeCSHandle mch,
M_ACL *acl
);
The ACL is not copied: the pointer must remain valid. The initial state is that no ACL is set for shares; to return to this state, pass a null pointer.
It is best to avoid using this function; instead, pass appropriate CardSet_flags_ to NFKM_makecardset_begin and it will construct and use an appropriate ACL.
|
NFKM_newkey_*
NFKM_newkey_makeacl
This function creates the ACL for a new key:
M_Status NFKM_newkey_makeacl(
NFast_AppHandle app,
NFastApp_Connection conn,
const NFKM_WorldInfo *world
const NFKM_CardSet *cardset
M_Word flags,
M_Word opperms_base,
M_Word opperms_maskout,
M_ACL *acl
struct NFast_Call_Context *cctx
);
-
const NFKM_WorldInfo *world
must be non-NULL
. -
const NFKM_CardSet *cardset
must beNULL
for module-only protection, or non-NULL
for Operator Card Set protection. -
The following
flags
are defined:-
NFKM_NKF_IKWID
If this flag is set,
NFKM_makeacl
does not perform its standard checks. This lets you create keys with esoteric ACLs.IKWID
stands for 'I know what I’m doing'. You should not set this flag unless you are sure this is true. -
NFKM_NKF_NVMemBlob
If this flag is set,
NFKM_makeacl
creates an NVRAM key blob, using the standard ACL options. -
NFKM_NKF_NVMemBlobX
If this flag is set,
NFKM_makeacl
creates an NVRAM key blob, using the extended options. -
NFKM_NKF_PerAuthUseLimit
If this flag is set,
NFKM_makeacl
creates an ACL with a per auth use limit. -
NFKM_NKF_Protection_mask
-
NFKM_NKF_ProtectionCardSet
-
NFKM_NKF_ProtectionModule
It is not necessary to set this flag in conjunction with
NFKM_makeacl
orNFKM_makeblobs
. -
NFKM_NKF_ProtectionNoKey
This flag can be used when generating only public keys.
-
NFKM_NKF_ProtectionUnknown
It is not necessary to set this flag in conjunction with
NFKM_makeacl
orNFKM_makeblobs
. -
NFKM_NKF_PublicKey
If this flag is set,
NFKM_makeacl
creates the ACL for the public half of a key. -
NFKM_NKF_Recovery_mask
-
NFKM_NKF_RecoveryDefault
,NFKM_NKF_RecoveryRequired
,NFKM_NKF_RecoveryDisabled
,NFKM_NKF_RecoveryForbidden
If any of these flags are returned by
NFKM_findkey
, it indicates that recovery is enabled.Result for a new key if the Security World has recovery: enabled disabled NFKM_NKF_RecoveryDefault
enabled
disabled
NFKM_NKF_RecoveryRequired
enabled
InvalidACL
NFKM_NKF_RecoveryDisabled
disabled
disabled
NFKM_NKF_RecoveryForbidden
InvalidACL
disabled
-
NFKM_NKF_RecoveryNoKey
If this flag is returned by
NFKM_findkey
, it indicates that there is no private key. -
NFKM_NKF_RecoveryUnknown
If this flag is returned by
NFKM_findkey
, it indicates that recovery is unknown. -
NFKM_NKF_SEEAppKey
If this flag is set,
NFKM_makeacl
creates an ACL with a certifier for a SEE World. It has been superseded byNFKM_NKF_SEEAppKeyHashAndMech
. -
NFKM_NKF_SEEAppKeyHashAndMech
If this flag is set,
NFKM_makeacl
creates an ACL with a certifier for a SEE World specifying the key hash and signing mechanism. -
NFKM_NKF_TimeLimit
If this flag is set,
NFKM_makeacl
creates an ACL with a time limit. -
NFKM_NKF_HasCertificate
-
-
M_ACL *acl
—the ACL will be overwritten and, therefore, should not contain any pointers to memory that has been operated on bymalloc
.Set to have
oppermissions
values like_Sign
,_Decrypt
,_UseAsBlobKey
,_UseAsCertificate
, or similar. In many cases, you can setoppermissions
to be one or more of the following macros, depending on the capabilities of the key:-
NFKM_DEFOPPERMS_SIGN
-
NFKM_DEFOPPERMS_VERIFY
-
NFKM_DEFOPPERMS_ENCRYPT
-
NFKM_DEFOPPERMS_DECRYPT
You can also use some combination of those macros for keys that can do both, such as RSA and symmetric keys:
#define NFKM_DEFOPPERMS_SIGN (Act_OpPermissions_Details_perms_Sign|Act_OpPermissions_Details_perms_UseAsCertificate |Act_OpPermissions_Details_perms_SignModuleCert) #define NFKM_DEFOPPERMS_VERIFY (Act_OpPermissions_Details_perms_Verify) #define NFKM_DEFOPPERMS_ENCRYPT (Act_OpPermissions_Details_perms_Encrypt|Act_OpPermissions_Details_perms_UseAsBlobKey) #define NFKM_DEFOPPERMS_DECRYPT (Act_OpPermissions_Details_perms_Decrypt|Act_OpPermissions_Details_perms_UseAsBlobKey)
If you wish to modify the default ACL, you may do so after calling this function. In such a case, the ACL will be allocated dynamically.
The
Protection
flags either must beUnknown
or they must beNFKM_Module
orNFKM_CardSet
and correspond to whethercardset
is non-NULL
. In any case,NFKM_CardSet
determines the protection.You must free the ACL at some point, either by using
NFastApp_FreeACL
or, if the ACL was part of a command, as part of a call toNFastApp_Free_Command
. -
NFKM_newkey_makeaclx
This is an alternative to NFKM_newkey_makeacl
which enables you to define more complex ACLs by defining input in the NFKM_MakeACLParams structures
.
M_Status NFKM_newkey_makeaclx(
NFast_AppHandle app,
NFastApp_Connection conn,
const NFKM_WorldInfo *w,
const NFKM_MakeACLParams *map,
M_ACL *acl,
struct NFast_Call_Context *cc
);
typedef struct NFKM_MakeACLParams {
M_Word f;
M_Word op_base, op_bic;
const NFKM_CardSet *cs;
const M_Hash *seeinteg; SEEAppKey
M_Word timelimit; TimeLimit
const M_KeyHashAndMech *seeintegkham; SEEAppKeyHashAndMech
M_Word pa_uselimit; PerAuthUseLimit
NFKM_FIPS140AuthHandle fips; NVMemBlob, maybe others later
const M_Hash *hknvacl; NVMemBlobX
} NFKM_MakeACLParams;
The values for NFKM_WorldInfo
and NFKM_CardSet
are the same as for NFKM_newkeymakeacl
.
If you are creating a key for a SEE application, specify the application signing key using a M_KeyHashAndMech . Use of an M_Hash is deprecated.
|
NFKM_newkey_makeblobs
This function creates the working and recovery blobs for a newly generated key:
M_Status NFKM_newkey_makeblobs(
NFast_AppHandle app,
const NFKM_WorldInfo *world,
M_KeyID privatekey,
M_KeyID publickey,
const NFKM_CardSet *cardset,
M_KeyID logtokenid,
M_Word flags,
NFKM_Key *newkeydata_io,
struct NFast_Call_Context *cctx
);
-
world
must be non-NULL. -
One or both of
privatekey
andpublickey
may be 0 if only one-half, or possibly even neither, is to be recorded. If the key is a symmetric key, supply it asprivatekey
. -
cardset
andlogtokenid
must be set consistently; either both must beNULL
or both must be non-NULL
, depending on whethercardset
was 0 inNFKM_makeacl
. -
flags
should be as inNFKM_makeacl
for the private half (_PublicKey
must not be specified).
This call overwrites the previous contents of newkeydata_io
members privblob
, -pubblob
and privblobrecov
, so these should not contain pointers to any memory that has been operated on by malloc
. This call also fills in the hash
member.
It does not change the other members, which must be set appropriately before the caller uses NFKM_recordkey
.
NFKM_newkey_makeblobsx
This function creates the working and recovery blobs for a newly generated key— it offers more functionality than NFKM_newkey_makeblobs
as you can specify details for the blobs in a parameters structure.
In particular it may be used to create a key blob stored in NVRAM.
M_Status NFKM_newkey_makeblobsx(
NFast_AppHandle app,
NFastApp_Connection conn,
const NFKM_WorldInfo *w,
const NFKM_MakeBlobsParams *mbp,
NFKM_Key *k,
struct NFast_Call_Context *cc
);
typedef struct NFKM_MakeBlobsParams {
M_Word f;
M_KeyID kpriv, kpub, lt;
const NFKM_CardSet *cs;
NFKM_FIPS140AuthHandle fips; NVMemBlob, maybe others later
M_KeyID knv; NVMemBlob[X]
M_KeyID knvacl; NVMemBlobX
} NFKM_MakeBlobsParams;
NFKM_newkey_writecert
Sets up the key generation certificate information for a new key.
M_Status NFKM_newkey_writecert(
NFast_AppHandle app,
NFastApp_Connection conn,
const NFKM_ModuleInfo *m,
M_KeyID kpriv,
M_ModuleCert *mc,
NFKM_Key *k,
struct NFast_Call_Context *cctx
);
The argument mc
should be the key generation certificate for a symmetric or private key.
To free the data stored in the Key structure, call NFKM_freecert
.
NFKM_operatorcard_changepp
This function has been superseded by the NFKM_changepp function, see NFKM_changepp.
|
This function changes the passphrase on an operator card:
M_Status NFKM_operatorcard_changepp(
NFast_AppHandle app,
NFastApp_Connection conn,
const NFKM_SlotInfo *slot,
const M_Hash *oldpp,
const M_Hash *newpp,
struct NFast_Call_Context *cctx
);
Either oldpp
or newpp
may be NULL
to indicate the absence of a passphrase.
NFKM_operatorcard_checkpp
This function has been superseded by the NFKM_checkpp function, see NFKM_checkpp.
|
This function checks the passphrase on an operator card:
M_Status NFKM_operatorcard_checkpp(
NFast_AppHandle app,
NFastApp_Connection conn,
const NFKM_SlotInfo *slot,
const M_Hash *pp,
struct NFast_Call_Context *cctx
);
pp
may be NULL
to indicate the absence of a passphrase.
NFKM_recordkey
This function writes the key blobs to the kmdata area of the host computer’s hard disk:
M_Status NFKM_recordkey(
NFast_AppHandle app,
NFKM_Key *key,
struct NFast_Call_Context *cctx
);
NFKM_recordkey
does not take over any of the memory in the key.
Whether the key is module protected, smart-card protected, or has some other kind of protection is inferred from the privblob
details.
The NFKM_Key
block should be cleared to all-bits-zero before use.
If you use any advanced features, set the version field (member v
) to the correct value before calling recordkey
.
NFKM_recordkeys
NFKM_recordkeys does the same job as NFKM_recordkey for multiple keys.
M_Status NFKM_recordkeys(
NFast_AppHandle app,
NFKM_Key **k,
size_t n,
struct NFast_Call_Context *cc
);
Either all the keys are written or none are.
NFKM_replaceacs_*
NFKM_replaceacs_abort
Destroys an admin card replacement context.
void NFKM_replaceacs_abort(
NFKM_ReplaceACSHandle rah
);
NFKM_ReplaceACSHandle rah
is the job handle returned by NFKM_replaceacs_begin
NFKM_replaceacs_begin
Starts a job to replace the Administrator Card Set.
The following diagram illustrates the paths through the NFKM_replaceacs
process:
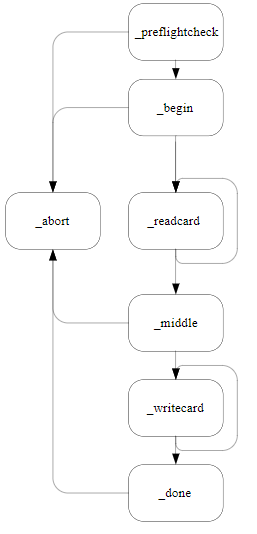
M_Status NFKM_replaceacs_begin(
NFast_AppHandle app,
NFastApp_Connection conn,
NFKM_ReplaceACSHandle *rah,
const NFKM_ModuleInfo *m,
struct NFast_Call_Context *cc
);
-
NFKM_ReplaceACSHandle *rah
is a pointer to the address to which the function will write the job handle -
const NFKM_ModuleInfo *m
is a pointer to the module to use for the transfer
If this function fails, there is nothing else to do; if it succeeds, you must either go all the way through NFKM_replaceacs_done
or call NFKM_replaceacs_abort
to throw away all of the state.
NFKM_replaceacs_done
Wraps up an admin card replacement job.
M_Status NFKM_replaceacs_done(
NFKM_ReplaceACSHandle rah
);
-
NFKM_ReplaceACSHandle rah
is the job handle returned byNFKM_replaceacs_begin
NFKM_replaceacs_gethash
Fetches the identifying hash for new administrator cards created by this job.
void NFKM_replaceacs_gethash(
NFKM_ReplaceACSHandle rah,
M_Hash *hh
);
-
NFKM_ReplaceACSHandle rah
is the job handle returned byNFKM_replaceacs_begin
-
M_Hash *hh
is a pointer to the address to write the hash
NFKM_replaceacs_middle
Does the work in the middle of an admin card set replacement job.
M_Status NFKM_replaceacs_middle(
NFKM_ReplaceACSHandle rah
);
NFKM_ReplaceACSHandle rah
is the job handle returned by NFKM_replaceacs_begin
NFKM_replaceacs_preflightcheck
Verifies that a replaceacs
operation is safe.
int NFKM_replaceacs_preflightcheck(
NFast_AppHandle app,
const NFKM_WorldInfo *w,
int *unsafe,
struct NFast_Call_Context *cc
);
-
NFKM_ReplaceACSHandle rah
is the job handle returned byNFKM_replaceacs_begin
-
const NFKM_WorldInfo *w
is a pointer to the world information -
int *unsafe
is cleared if safe, nonzero if not
If the operation is safe, *unsafe
is cleared; otherwise it will contain a nonzero value.
Later, this might explain in more detail what the problem is.
Currently, the only check is for world file entries which aren’t understood (and therefore might be blobs of keys which would need to be replaced).
NFKM_replaceacs_readcard
Reads an administrator card, with a view to replacing it.
M_Status NFKM_replaceacs_readcard(
NFKM_ReplaceACSHandle rah,
const NFKM_SlotInfo *s,
const M_Hash *pp,
int *left
);
-
NFKM_ReplaceACSHandle rah
is the job handle returned byNFKM_replaceacs_begin
-
const NFKM_SlotInfo *s
is a pointer to the slot containing the admin card -
const M_Hash *pp
is a pointer to the passphrase hash for the card -
int *left
is a pointer to the address to store number of cards remaining
NFKM_replaceacs_writecard
Writes a replacement administrator card.
M_Status NFKM_replaceacs_writecard(
NFKM_ReplaceACSHandle rah,
NFKM_SlotInfo *s, const M_Hash *pp,
int *left
);
-
NFKM_ReplaceACSHandle rah
is the job handle returned byNFKM_replaceacs_begin
-
NFKM_SlotInfo *s
is a pointer to the slot containing admin card -
const M_Hash *pp
is a pointer to the passphrase hash for the card -
int *left
is a pointer to the address to store number of cards remaining